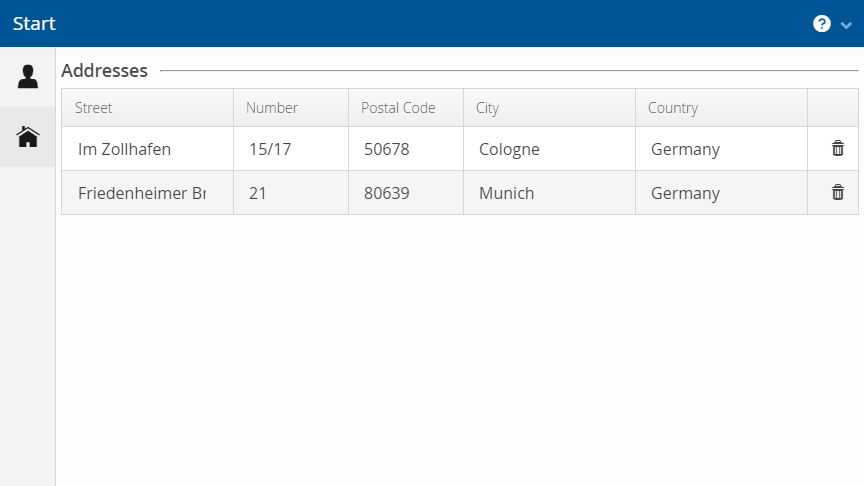
Step 5: Adding delete buttons
The next step is to add delete buttons to the rows containing the address data on the address page, so that the user is able to delete addresses.
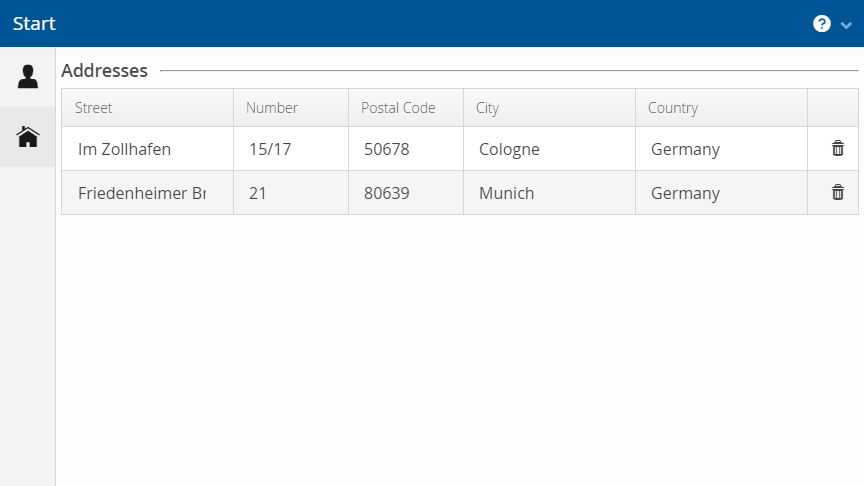
Figure 1. Address table with delete buttons
These buttons can be implemented in the PMO class. We simply define a new @UIButton
and pass the element we want to delete to a consumer in its method. This consumer is stored as a new attribute in the AddressRowPmo
and AddressTablePmo
classes and is set at construction.
AddressRowPmo.java
private Address address;
private Consumer<Address> deleteConsumer;
public AddressRowPmo(Address address, Consumer<Address> deleteConsumer) {
this.address = address;
this.deleteConsumer = deleteConsumer;
}
@ModelObject
public Address getAddress() {
return address;
}
...
@UITableColumn(width = 50)
@UIButton(position = 60, captionType = CaptionType.NONE, showIcon = true,
icon = VaadinIcons.TRASH)
public void deleteButton() {
deleteConsumer.accept(address);
}
In the AddressPage
, we implement the actual method that deletes the address for the particular partner and use it as the Consumer
when instantiating AddressTablePmo
.
AddressPage.java
public AddressPage(
BusinessPartner partner, BusinessPartnerRepository repository) {
this.partner = partner;
this.addressTable = new AddressTablePmo(partner, this::deleteAddress);
this.repository = repository;
}
public void deleteAddress(Address address) {
partner.removeAddress(address);
}