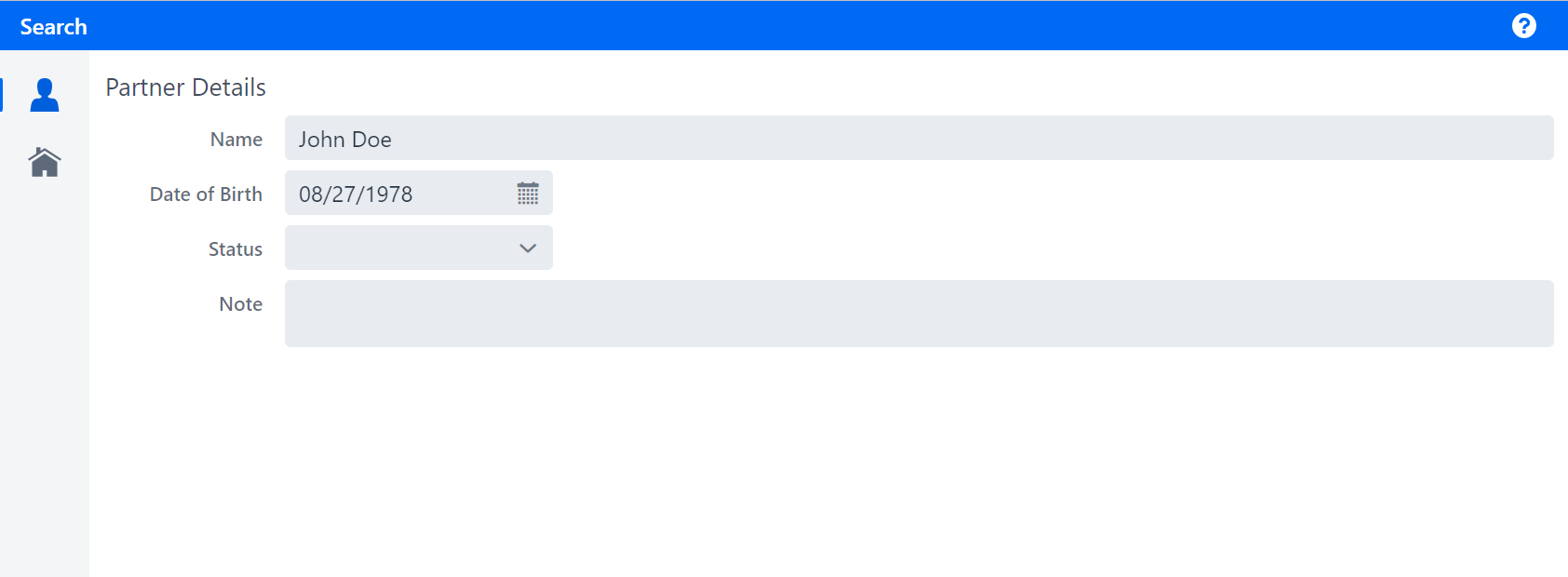
Step 5: UIDateField, UIComboBox & UITextArea
This step introduces you to the UI elements UIDateField , UIComboBox and UITextArea .
|
Next, you will create and fill out a page to display general information of a business partner, such as the name and date of birth.
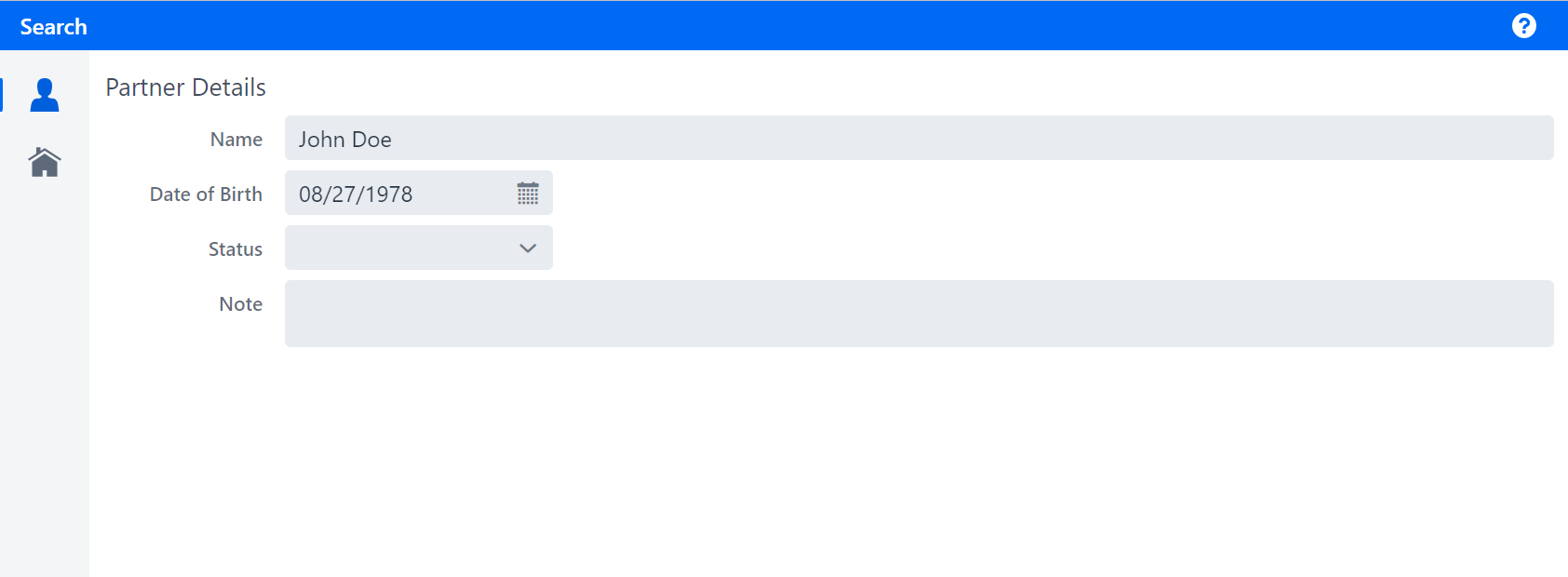
Implementing a new PMO
The page should contain a section to display partner details. Therefore, create a new class PartnerDetailsSectionPmo
and annotate it with @UISection
. Its caption should be set to "Partner Details".
PartnerDetailsSectionPmo
should define UI Elements for the name and date of birth of the partner as well as their status and some notes. You need a UITextField
, a UIDateField
, a UIComboBox
and a UITextArea
. Implement them as follows:
-
Pass a
BusinessPartner
to the constructor and assign it to the fieldpartner
. -
Create and implement the getter method for the property
name
and annotate it with@UITextField
.-
Get the name by calling the getter method on the partner.
-
The label should be set to "Name".
-
-
Create and implement the setter method for the property
name
. Set the name by calling the setter method on the partner. -
Create and implement the getter method for the property
dateOfBirth
with the signatureLocalDate getDateOfBirth()
the same way as in 2i. -
Annotate the getter with
@UIDateField
. The label should be set toDate of Birth
. -
Create and implement the setter method for the property
dateOfBirth
the same way as in 3. -
Create and implement the getter method for the property
status
with the signatureStatus getStatus()
. -
Annotate the getter with
@UIComboBox
. The label should be set to "Status". -
Create and implement the setter method for the property
status
. -
Create and implement the getter method for the property
note
with the signatureString getNote()
. -
Annotate the getter with
@UITextArea
. The label should be set to "Note". -
Create and implement the setter method for the property
note
.
The finished implementation should look like this:
@UISection(caption = "Partner Details")
public class PartnerDetailsSectionPmo {
private final BusinessPartner partner;
public PartnerDetailsSectionPmo(BusinessPartner partner) {
this.partner = partner;
}
@UITextField(position = 10, label = "Name")
public String getName() {
return partner.getName();
}
public void setName(String name) {
partner.setName(name);
}
@UIDateField(position = 20, label = "Date of Birth")
public LocalDate getDateOfBirth() {
return partner.getDateOfBirth();
}
public void setDateOfBirth(LocalDate dateOfBirth) {
partner.setDateOfBirth(dateOfBirth);
}
@UIComboBox(position = 30, label = "Status")
public Status getStatus() {
return partner.getStatus();
}
public void setStatus(Status status) {
partner.setStatus(status);
}
@UITextArea(position = 40, label = "Note")
public String getNote() {
return partner.getNote();
}
public void setNote(String note) {
partner.setNote(note);
}
Creating the basic data page
Now that the section is done, create a new AbstractPage
called BasicDataPage
, initialize it as you did in step 1 and add the PartnerDetailsSectionPmo
in the createContent
method.
Updating PartnerDetailsView
Since you have created the BasicDataPage
, you need to update PartnerDetailsView
. If you remember what was said in the first step of this tutorial, you know you have to call BasicDataPage.init()
after calling the constructor, otherwise the content of the page won’t be created.
Therefore, update the method createBasicDataPage
as follows:
-
Create a new instance of
BasicDataPage
. -
Call the
init()
method. -
Return the created
BasicDataPage
.
The finished implementation should look like this:
private Component createBasicDataPage(BusinessPartner partner) {
BasicDataPage basicDataPage = new BasicDataPage(partner);
basicDataPage.init();
return basicDataPage;
}
You should now be able to see a BasicDataPage
with the personal information of a partner. For John Doe, you should only be able to see their name and date of birth (27/08/1978).
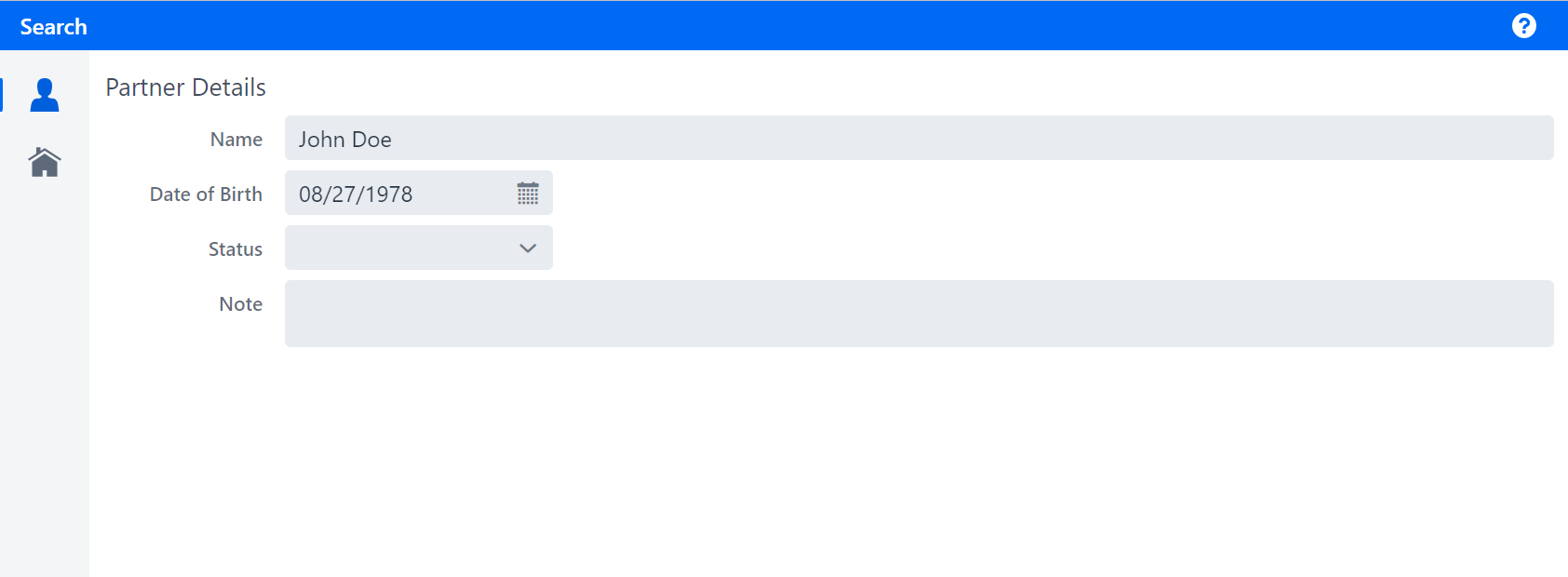
The next step extends the UI to display all addresses of a partner.